Call Lua Script in Visual C++ / MFC Sample
*Lua script
(below) to customize preferences and content of
UI (above).
1
This article shows a way to integrate Lua in
your application.
2
Lua is
an extension programming language designed to
support general procedural programming with data
description facilities. It also offers good
support for object-oriented programming,
functional programming, and data-driven
programming. Lua is
intended to be used as a powerful, light-weight
configuration language for any program that
needs one. Lua is
implemented as a library, written in clean C
(that is, a common subset of ANSI C and
C++).
Sample of Lua Syntax
(FOR loop):
Collapse | Copy
Code
for i=1,10 do
-- the first program in every language
io.write("Hello world, from ",_VERSION,"!\n")
end
-
For full description see: About.
3
This sample is a WTL application (simple HTML
help systems), that integrates Lua scripts
to customize preferences and content.
It defines the Lua function:
Collapse | Copy
Code
-- # MessageBox
--------------------------------------------------------
-- int MessageBox(
-- string msg, |= Message to display
-- string capition |= Capition of Box
-- );
-- Return Value:
-- if 1 the user click in OK or user close the box
-- # ShowContentPainel
-----------------------------------------------------------------
-- void ShowContentPainel(
-- bool bShow |= If true, the painel start opened, if false not.
-- );
-- # SetWindowStartSize
-----------------------------------------------------------------
-- void SetWindowStartSize(
-- number w, |= Start W size of window
-- number h, |= Start H size of window
-- );
-- Remarks:
-- if this function is not called, the default size is 800 x 600
-- # SetMinWindowSize
-----------------------------------------------------------------
-- void SetMinWindowSize(
-- number w, |= Minimum W size of window
-- number h, |= Minimum H size of window
-- );
-- # SetTitle
-----------------------------------------------------------------
-- void SetTitle(
-- string title |= Text that be title of window.
-- );
-- # Navigate
-----------------------------------------------------------------
-- void Navigate(
-- string url |= Url
-- );
-- # InsertItemInPainel
-----------------------------------------------------------------
-- void InsertItemInPainel(
-- string title, |= Text displayed in tree
-- string url, |= Url
-- number icon, |= Icon of item, the possible values
---are: 0 = BOOK, 1 = FILE, 2 = NETFILE
-- number id, |= Id of item, this has to be unique and start in 1
-- number idp |= Parent item, this is a ID of a item that is
---the parent or '0' for root item.
-- );
-- sample:
-- ICON BOOK / ID 1 / In ROOT
-- InsertItemInPainel("Trinity Systems",
"http://www.novaamerica.net/trinitysystems/", 0, 1, 0);
-- ICON NETFILE / ID 2 / In ID1 (Trinity Systems)
-- InsertItemInPainel("Orion",
"http://www.novaamerica.net/trinitysystems/Orion", 2, 2, 1);
-- # ExpandItemInPainel
------------------------------------------------------------------
-- void ExpandItemInPainel(
-- string id |= Id of item
-- );
-- Remarks:
-- This function need to be called after InsertItemInPainel's
...and now I'll show you how to create these
functions in Lua/C++.
4
-
The first thing to do is to
build the DLL that contains Lua.
(download Lua DLL
demo project)
-
Link this in your project:
Collapse | Copy
Code
#if defined (_DEBUG)
#pragma comment( lib, "lua.lib" ) #else
#pragma comment( lib, "lua.lib" ) #endif
Remember: To change your
Project Property -> linker -> general ->
additional library directory: lua lib
directory.
-
Add Lua include
files:
Collapse | Copy
Code
extern "C"
{
#include <span class="code-string">"lua.h"
</span>
}
Remember: To change your
Project Property -> C/C++
-> general -> additional include
directories: lua include
directory.
Notice that all the files in lua lib
need to stay with "c" extension, because Lua was
written to be ANSI C compliant.
-
Now we need to start Lua VM
as follows:
Collapse | Copy
Code
LRESULT OnCreate(UINT
, WPARAM , LPARAM , BOOL&
)
{
lua_State *luaVM = lua_open();
luaopen_base(luaVM );
luaopen_table(luaVM );
luaopen_io(luaVM );
luaopen_string(luaVM );
luaopen_math(luaVM );
if (NULL ==
luaVM)
{
MessageBox("Error Initializing lua\n");
}
code.
lua_close(luaVM);
End
}
-
Now we make a glue function
of Lua and
C/C++.
The Lua API
function to do this is:
Collapse | Copy
Code
lua_register(s, n, g)
where
-
s :
is the lua_State to
register the function.
-
n :
is the name of the function exposed to Lua.
-
g :
the C/C++ glue function.
See the sample:
Collapse | Copy
Code
lua code.
lua_register( luaVM, "SetHome", l_SetHome );
-------------------------------------------
#Lua Functions
------------------------------------------
static
int l_SetTitle( lua_State*
luaVM)
{
const char*
title = luaL_checkstring(luaVM,
1);
theMainFrame->SetWindowText(title);
return 0;
}
-
Now we need the load and
execute Lua scripts:
Collapse | Copy
Code
code.
lua_register( luaVM, "SetHome", l_SetHome );
functions
lua_dofile(luaVM, "hrconf.lua");
The Lua API
function to do this is:
Collapse | Copy
Code
lua_dofile(s, p)
where
-
s :
is the lua_State to
register the function.
-
p :
path for Lua script
file.
See the demo script.
For a complete understanding of the Lua API,
see: Lua 5.0
Reference Manual.
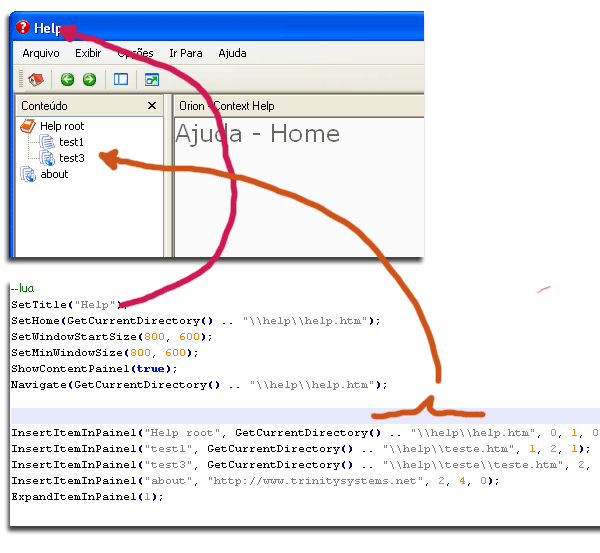
4
Lua_Lib.zip
News:
1 UCanCode Advance E-XD++
CAD Drawing and Printing Solution
Source Code Solution for C/C++, .NET V2025 is released!
2
UCanCode Advance E-XD++
HMI & SCADA Source Code Solution for C/C++, .NET V2025 is released!
3
UCanCode
Advance E-XD++ GIS SVG Drawing and Printing Solution
Source Code Solution for C/C++, .NET V2025 is released!
Contact UCanCode Software
To buy the source code or learn more about with:
Next-->
Promotional personalized database
document printing Solution
|
|